Landing Page Testimonial List Component
- Updated on
Use this component to display a list of testimonials. Each testimonial has text, a name, and a picture of the person.
Testimonials are a great way to show that other people have used your product and are happy with it. Consider adding it high up on your landing page.
Shorter testimonials can be made smaller by setting the size
prop to half
or third
. The default size is full
.
Don't take it from us
See what other people have to say.
“This is the best thing since sliced bread. I cannot believe I did not think of it myself.”
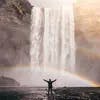
“After using this, I cannot imagine going back to the old way of doing things.”

“Perfect for my use case”
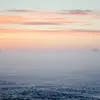
“Excellent product!”
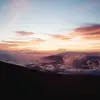
“Can easily recommend!”
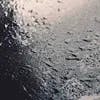
Usage
import { LandingTestimonialListSection } from '@/components/landing/testimonial/LandingTestimonialList';
const testimonialItems = [
{
name: 'Parl Coppa',
text: 'This is the best thing since sliced bread. I cannot believe I did not think of it myself.',
handle: '@coppalipse',
imageSrc: 'https://picsum.photos/100/100.webp?random=1',
size: 'half',
},
{
name: 'Mathew',
text: 'After using this, I cannot imagine going back to the old way of doing things.',
handle: '@heymatt_oo',
imageSrc: 'https://picsum.photos/100/100.webp?random=2',
size: 'half',
},
{
name: 'Joshua',
text: 'Perfect for my use case',
handle: '@joshua',
imageSrc: 'https://picsum.photos/100/100.webp?random=3',
size: 'third',
},
{
name: 'Mandy',
text: 'Excellent product!',
handle: '@mandy',
imageSrc: 'https://picsum.photos/100/100.webp?random=4',
size: 'third',
},
{
name: 'Alex',
text: 'Can easily recommend!',
handle: '@alex',
imageSrc: 'https://picsum.photos/100/100.webp?random=5',
size: 'third',
},
];
<LandingTestimonialListSection
title="Don't take it from us"
description="See what other people have to say."
testimonialItems={testimonialItems}
/>
Examples
Background, links and features
This component supports different background colors.
Here we set variant to secondary.
Testimonials can also be linked + be featured and you can mix and match to send the desired message.
Don't take it from us
See what other people have to say.
“This is the best thing since sliced bread. I cannot believe I did not think of it myself.”
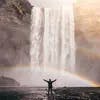
“After using this, I cannot imagine going back to the old way of doing things.”

“Perfect for my use case”
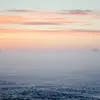
With Background Glow
Don't take it from us
See what other people have to say.
“This is the best thing since sliced bread. I cannot believe I did not think of it myself.”
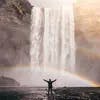
“After using this, I cannot imagine going back to the old way of doing things.”

“Perfect for my use case”
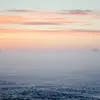
With Read More Wrapper
Don't take it from us
See what other people have to say.
“This is the best thing since sliced bread. I cannot believe I did not think of it myself.”
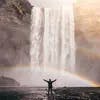
“After using this, I cannot imagine going back to the old way of doing things.”

“Perfect for my use case”
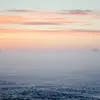
API Reference
Prop Name | Prop Type | Required | Default |
---|---|---|---|
title | string ǀ React.ReactNode | No | - |
titleComponent | React.ReactNode | No | - |
description | string ǀ React.ReactNode | No | - |
descriptionComponent | React.ReactNode | No | - |
testimonialItems | TestimonialItem[] | Yes | - |
withBackground | boolean | No | false |
variant | 'primary' ǀ 'secondary' | No | 'primary' |
withBackgroundGlow | boolean | No | false |
backgroundGlowVariant | 'primary' ǀ 'secondary' | No | 'primary' |
export interface TestimonialItem {
className?: string;
url?: string;
text: string;
imageSrc: string;
name: string;
handle: string;
featured?: boolean;
verified?: boolean;
size?: 'full' | 'half' | 'third'; // NB: Only applies to testimonials in a list, not grid.
}
More Examples
For more even more examples, see our Landing Page Component Examples page or see complete landing page examples by Exploring Our Landing Page Templates.