Blog Post
- Updated on
The LandingBlogPost
component displays a single blog post card with an image, author information, date, title, summary, reading time, and tags. It's designed to be used within a blog list component but can also function as a standalone element.
Can be used in a LandingBlogList
component or as a standalone element.
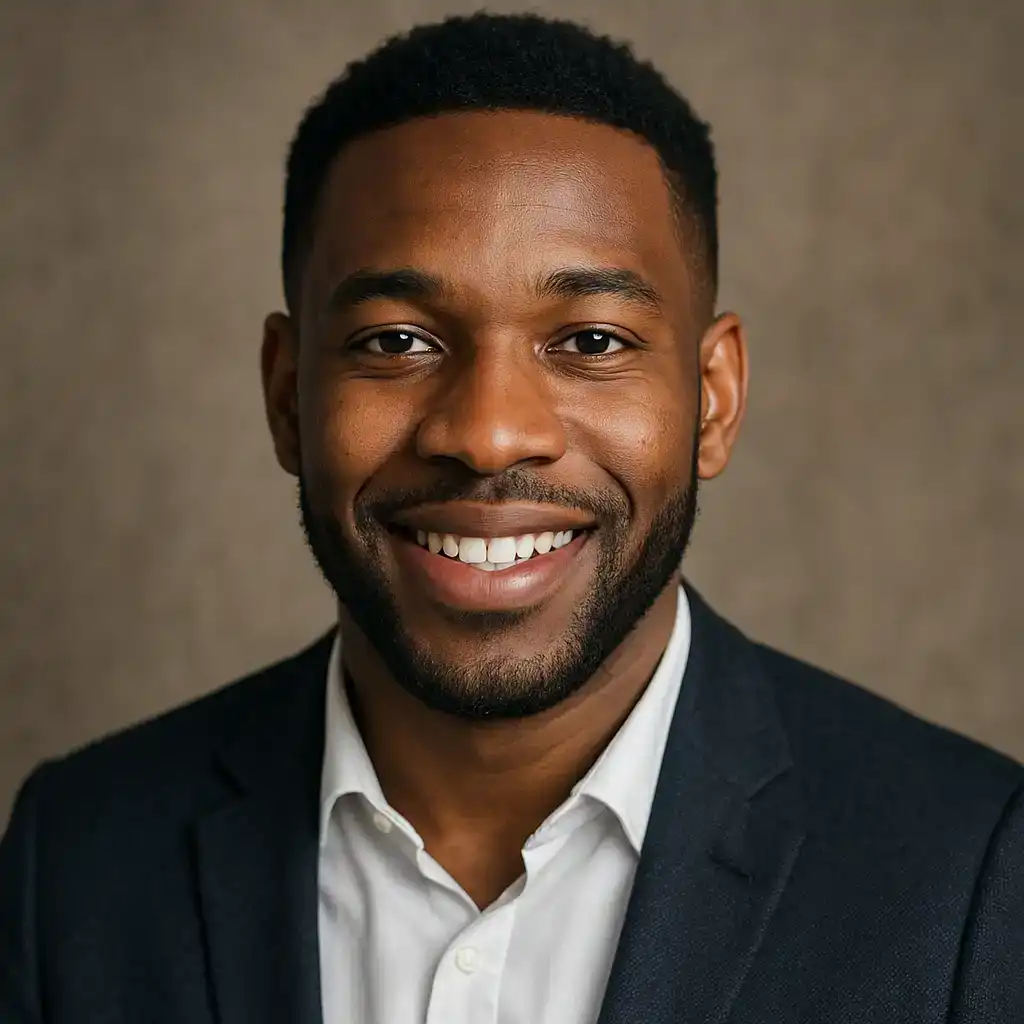
Getting Started with Shipixen
Learn how to quickly set up and customize your Shipixen landing page with our comprehensive guide.
Usage
Import the component and provide a post object with the required properties:
import { LandingBlogPost } from '@/components/landing/blog/LandingBlogPost';
<LandingBlogPost
post={{
slug: 'blog-post-slug',
date: 'November 15, 2023',
title: 'Blog Post Title',
summary: 'This is a summary of the blog post content.',
tags: ['Tag 1', 'Tag 2'],
images: ['/static/images/backdrop-1.webp'],
readingTime: 5,
author: {
name: 'John Doe',
avatar: '/static/images/people/1.webp'
}
}}
/>
Examples
Basic Blog Post
A minimal blog post card with only the essential information:
Minimal Blog Post Example
This is a minimal blog post example with only the essential information.
Image Position Variants
The LandingBlogPost
component supports three image position layouts:
Left Image Layout
Blog Post with Left Image
This example shows a blog post with the image positioned on the left side.
Center Image Layout
Used by default in grid displays:
Blog Post with Center Image
This example shows a blog post with the image positioned at the top (center layout).
Right Image Layout (Default)
Used by default in list displays:
Blog Post with Right Image
This example shows a blog post with the image positioned on the right side.
With Image and Summary
A blog post card featuring an image and summary text:
Blog Post with Image and Summary
This is a brief summary of the blog post that gives readers an idea of what to expect.
With Author and Reading Time
A blog post with author information and reading time displayed:
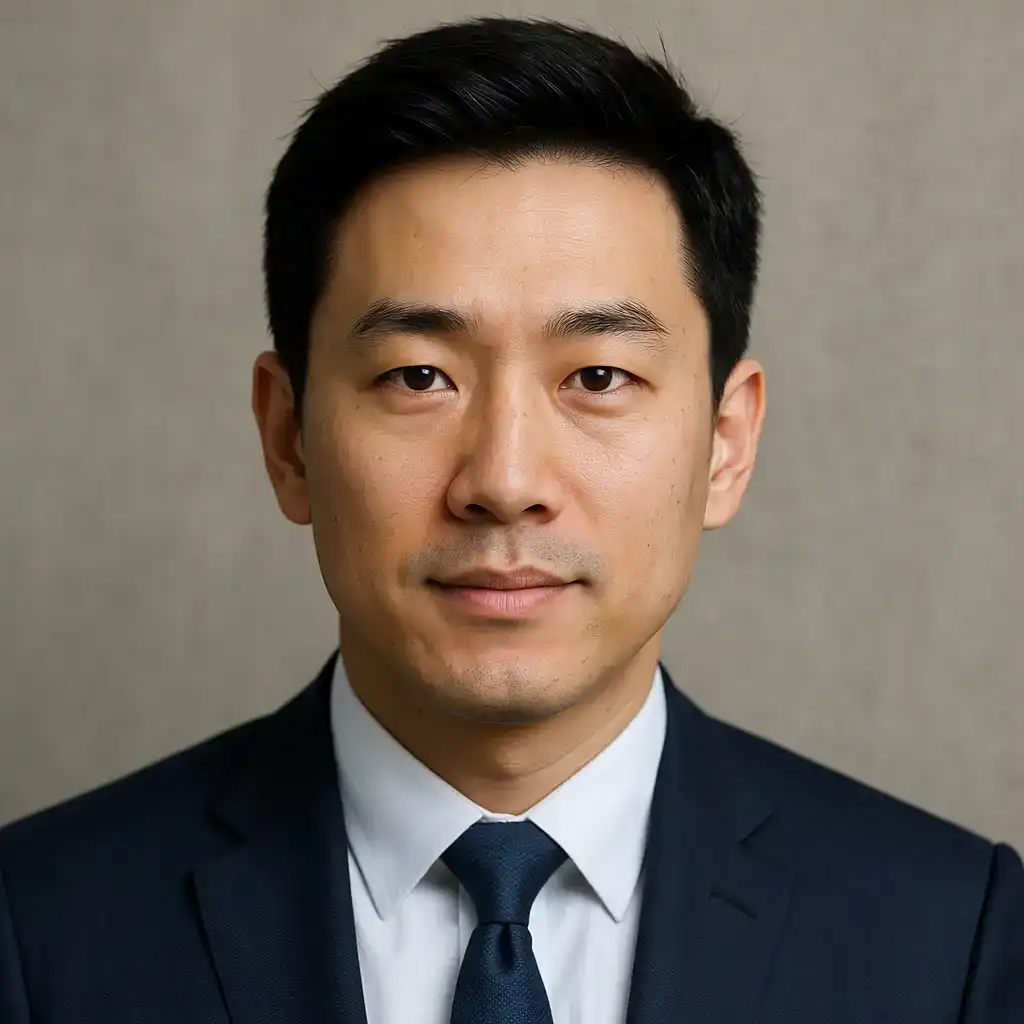
Blog Post with Author Information
A comprehensive guide written by an expert in the field.
With Tags
A blog post card displaying category tags:
Blog Post with Category Tags
Learn about the latest features and updates in our newest release.
With Clickable Tags
A blog post with tags that link to other pages:
Complete Example
A fully-featured blog post card with all available properties:
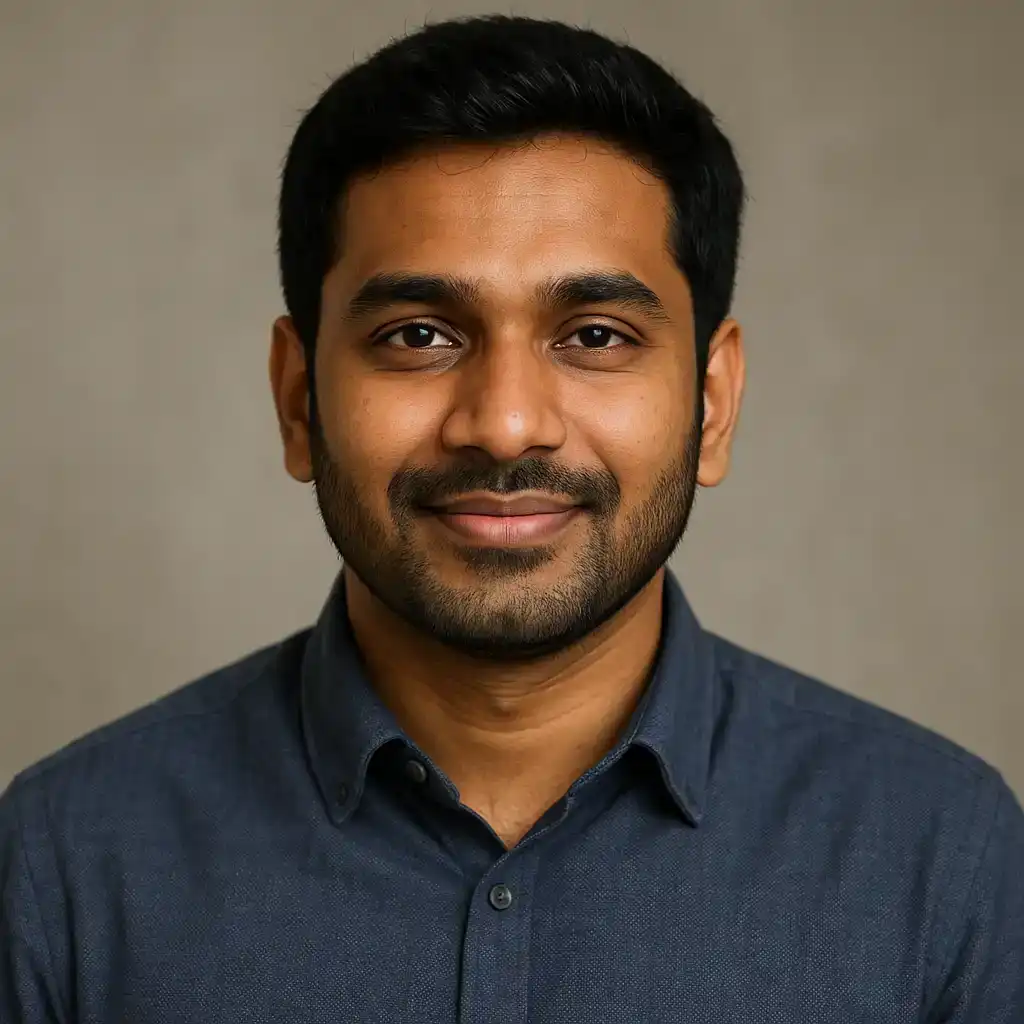
The Complete Guide to Modern Web Development
Explore the latest tools, frameworks, and best practices for building exceptional web experiences in 2023 and beyond.
API Reference
Prop Name | Prop Type | Required | Default |
---|---|---|---|
post | BlogPost | Yes | - |
imagePosition | 'left' | 'center' | 'right' | No | 'right' |
export interface BlogPost {
slug: string; // Unique identifier for the blog post
date: string; // Publication date of the post
title: string; // Title of the blog post
summary?: string; // Brief summary of the blog post content
tags?: string[] | Array<{url: string; text: string}>; // Array of category tags (strings) or objects with url and text properties
images?: string[]; // Array of image URLs, with the first one used as the post thumbnail
readingTime?: number; // Estimated reading time in minutes
author?: {
name?: string; // Name of the blog post author
avatar?: string; // URL to the author's avatar image
};
}
Usage with LandingBlogList
When using LandingBlogPost
inside a LandingBlogList
component, the imagePosition
depends on the list's display mode:
- For
display="grid"
(grid layout), theimagePosition
should be set to'center'
- For
display="list"
(list layout), theimagePosition
should be set to'right'
(default)
More Examples
For more examples, see Blog List.