Landing Page Testimonial Grid Component
- Updated on
Use this component to display a grid of testimonials.
This component accepts a title, description and a list of testimonials. They will be placed in a column layout on small screens, then a 2-column layout and finally a 3-column layout on large screens.
Each testimonial can be featured or not. The featured testimonial will stand out with bigger & bolder text.
Testimonials are a great way to show that other people have used your product and are happy with it. Consider adding it high up on your landing page.
Don't take it from us
See what other people have to say.
βAfter using this, I cannot imagine going back to the old way of doing things.β

βPerfect for my use caseβ
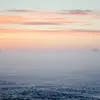
βThis is the best thing since sliced bread. I cannot believe I did not think of it myself.β
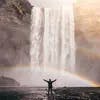
βExcellent product!β
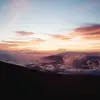
βCan easily recommend!β
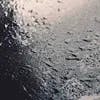
βI am very happy with the results.β
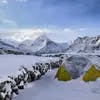
Usage
import { LandingTestimonialGrid } from '@/components/landing/testimonial/LandingTestimonialGrid';
const testimonialItems = [
{
name: 'Mathew',
text: 'After using this, I cannot imagine going back to the old way of doing things.',
handle: '@heymatt_oo',
imageSrc: 'https://picsum.photos/100/100.webp?random=2',
},
{
name: 'Joshua',
text: 'Perfect for my use case',
handle: '@joshua',
imageSrc: 'https://picsum.photos/100/100.webp?random=3',
},
{
name: 'Parl Coppa',
text: 'This is the best thing since sliced bread. I cannot believe I did not think of it myself.',
handle: '@coppalipse',
imageSrc: 'https://picsum.photos/100/100.webp?random=1',
featured: true, // Feature this testimonial
},
{
name: 'Mandy',
text: 'Excellent product!',
handle: '@mandy',
imageSrc: 'https://picsum.photos/100/100.webp?random=4',
},
{
name: 'Alex',
text: 'Can easily recommend!',
handle: '@alex',
imageSrc: 'https://picsum.photos/100/100.webp?random=5',
},
{
name: 'Sam',
text: 'I am very happy with the results.',
handle: '@sama',
imageSrc: 'https://picsum.photos/100/100.webp?random=6',
},
];
<LandingTestimonialGrid
title="Don't take it from us"
description="See what other people have to say."
testimonialItems={testimonialItems}
/>
Examples
Background, links and features
This component supports different background colors.
Here we set variant to secondary. Testimonials can also be linked + be featured and you can mix and match to send the desired message.
Don't take it from us
See what other people have to say.
βAfter using this, I cannot imagine going back to the old way of doing things.β

βPerfect for my use caseβ
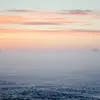
βThis is the best thing since sliced bread. I cannot believe I did not think of it myself.β
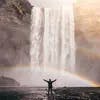
βExcellent product!β
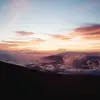
βCan easily recommend this product! I am very happy with the results.β
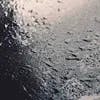
βI am very happy with the results.β
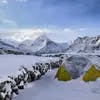
Background Glow
Don't take it from us
See what other people have to say.
βAfter using this, I cannot imagine going back to the old way of doing things.β

βPerfect for my use caseβ
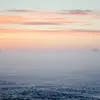
βThis is the best thing since sliced bread. I cannot believe I did not think of it myself.β
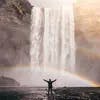
βExcellent product!β
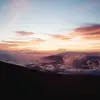
βCan easily recommend!β
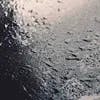
βI am very happy with the results.β
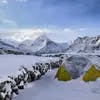
Read more wrapper
If your testimonials exceed 2 rows, you can add a "Read more" wrapper to hide the rest of the content initially.
This is usually a good idea to keep the page clean and focused.
Don't take it from us
See what other people have to say.
βAfter using this, I cannot imagine going back to the old way of doing things.β

βPerfect for my use caseβ
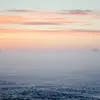
βThis is the best thing since sliced bread. I cannot believe I did not think of it myself.β
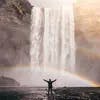
βExcellent product!β
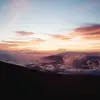
βCan easily recommend!β
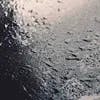
βI am very happy with the results.β
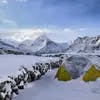
API Reference
Prop Name | Prop Type | Required | Default |
---|---|---|---|
title | string Η React.ReactNode | No | - |
description | string Η React.ReactNode | No | - |
testimonialItems | Array<TestimonialItem> | Yes | - |
featuredTestimonial | TestimonialItem | No | - |
withBackground | boolean | No | - |
variant | 'primary' Η 'secondary' | No | 'primary' |
withBackgroundGlow | boolean | No | false |
backgroundGlowVariant | 'primary' Η 'secondary' | No | 'primary' |
export interface TestimonialItem {
className?: string;
url?: string;
text: string;
imageSrc: string;
name: string;
handle: string;
featured?: boolean;
verified?: boolean;
size?: 'full' | 'half' | 'third'; // NB: Only applies to testimonials in a list, not grid.
}
More Examples
For more even more examples, see our Landing Page Component Examples page or see complete landing page examples by Exploring Our Landing Page Templates.