- Updated on
Ultimate Guide to Content Management in Next.js
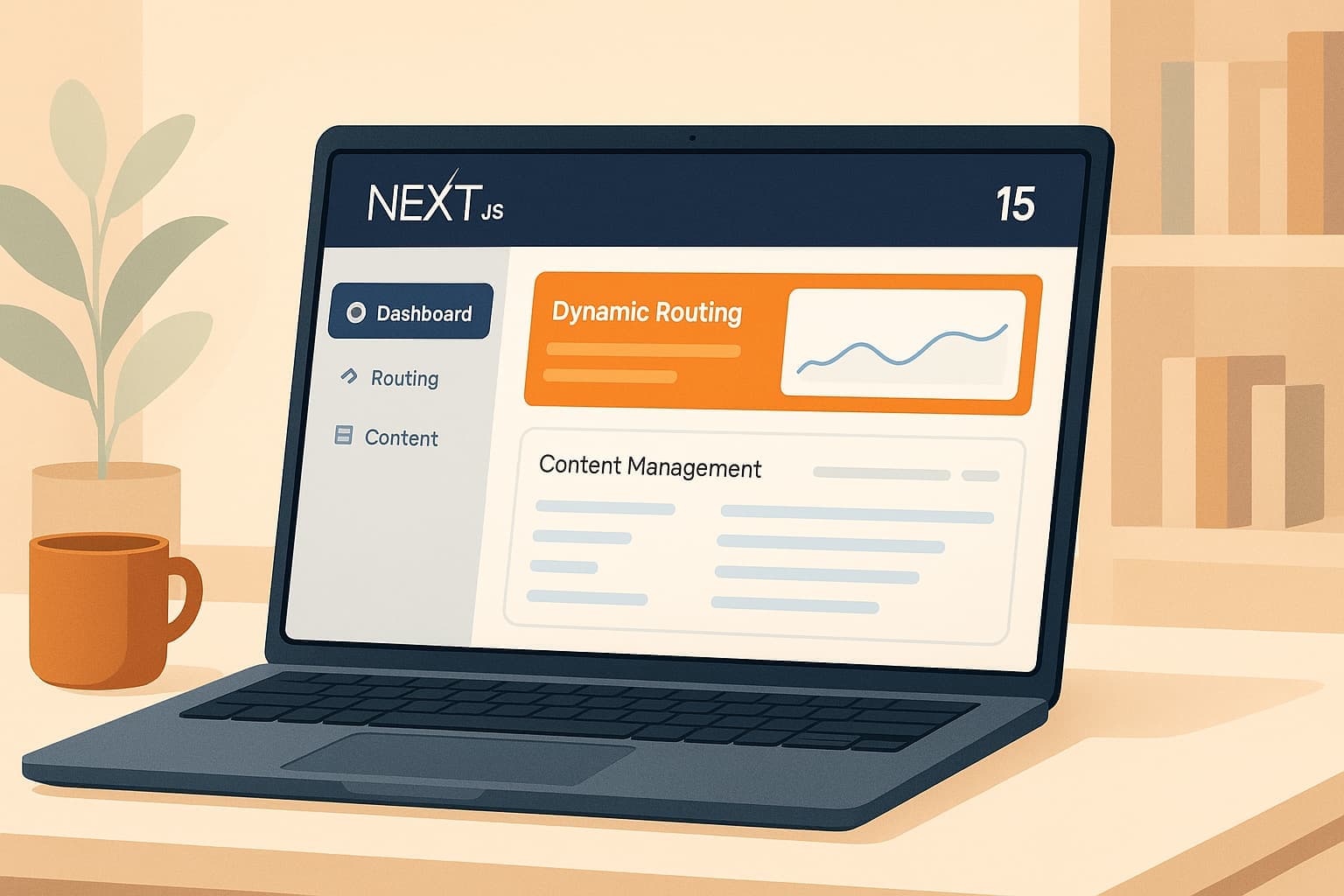
Managing content in Next.js has never been easier. With the release of Next.js 15, developers can combine Static Site Generation (SSG), Server-Side Rendering (SSR), and MDX support to create fast, dynamic, and SEO-friendly content. Here's what you need to know:
- Core Features: Next.js 15 includes tools like the App Router for dynamic routing, native MDX support for combining Markdown and React components, and improved static generation for performance.
- Content Organization: Use a well-structured project layout to separate components, content, and utilities for better scalability.
- Dynamic Content: Combine static MDX files with headless CMS integration for flexibility - ideal for blogs, documentation, and real-time updates.
- Reusable Components: Build modular UI and content blocks to save time and ensure consistency.
- SEO & Performance: Optimize metadata, caching, and accessibility to improve search rankings and user experience.
Quick Overview:
Feature | Benefit | Example Use Case |
---|---|---|
Static MDX | Fast loading, version control | Blogs, documentation |
Dynamic CMS | Real-time updates, collaboration | News, product pages |
Hybrid Content | Combines flexibility and control | E-commerce, landing pages |
By leveraging these tools and strategies, you can build a scalable, efficient, and user-friendly content management system in Next.js.
Next.js
Building Modern CMS Driven Web Applications with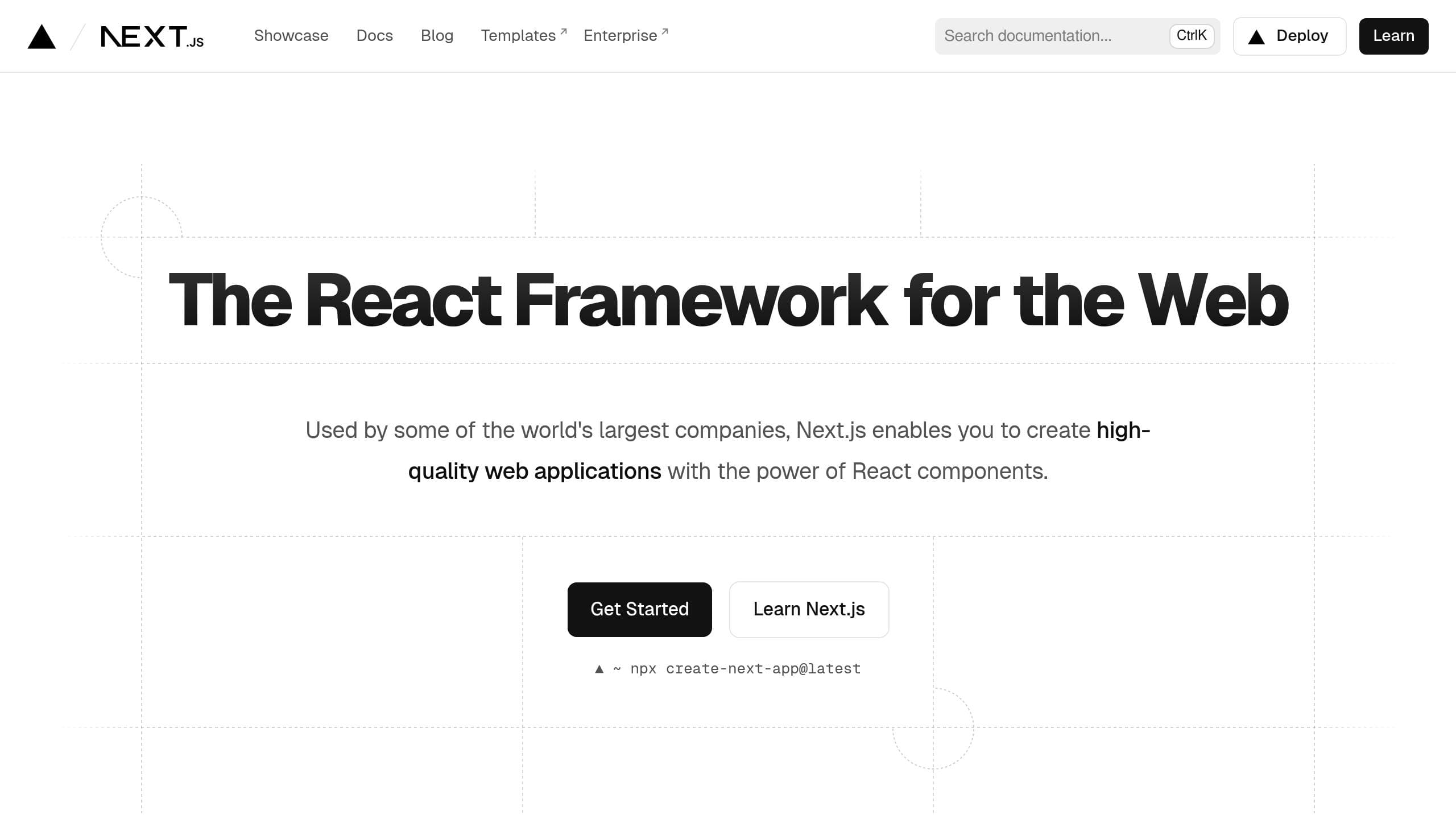
Project Structure for Content Management
A well-organized project structure simplifies content management in Next.js. Here's a suggested setup to help you keep things tidy and efficient.
File and Folder Organization
For content-focused Next.js apps, consider the following structure:
my-nextjs-project/
├── app/
│ ├── blog/
│ │ ├── [slug]/
│ │ │ └── page.tsx
│ │ └── page.tsx
│ └── layout.tsx
├── components/
│ ├── ui/
│ │ ├── button.tsx
│ │ └── card.tsx
│ └── content/
│ ├── blog-card.tsx
│ └── article-layout.tsx
├── content/
│ └── blog/
│ ├── post-1.mdx
│ └── post-2.mdx
└── lib/
└── content/
└── mdx-utils.ts
This structure separates concerns, making it easier to manage components, content, and utility functions.
Setting Up Dynamic Routes
Next.js 15 simplifies dynamic routing with folder-based configuration. Here's an example:
export default async function BlogPost({ params }) {
const { slug } = params;
const post = await getPostBySlug(slug);
return (
<article>
<h1>{post.title}</h1>
{post.content}
</article>
);
}
Dynamic routes allow you to load content dynamically based on the URL, making your app scalable and user-friendly.
Building Reusable Components
Reusable components are key to consistent and efficient development. Here's a quick breakdown:
Component Type | Purpose | Usage |
---|---|---|
UI Components | Basic interface elements | Use libraries like Shadcn UI for styling |
Landing Page Components | Landing page specific components | Use Page UI for faster development |
Content Blocks | Modular content sections | Create themed blocks with tools like Page UI |
Layout Components | Structure for pages | Build adaptable containers for your content |
For faster development, tools like Shipixen provide pre-designed, themed components that ensure visual consistency.
Key principles for your components:
- Component Isolation: Each component should handle a specific task or display function.
- Visual Consistency: Use shared design tokens to maintain a uniform look and feel.
- Content Adaptability: Design components to support various types of content.
Content Source Management
Organizing and retrieving content efficiently in Next.js is essential for building dynamic, scalable applications. Here's how you can handle various content types effectively.
Using MDX Files
MDX lets you combine Markdown with React components, offering a powerful way to create content. Tools like Contentlayer simplify managing MDX files. Here's an example of defining document types for MDX:
// lib/mdx-config.ts
import { defineDocumentType } from 'contentlayer/source-files'
export const Post = defineDocumentType(() => ({
name: 'Post',
filePathPattern: `posts/**/*.mdx`,
fields: {
title: { type: 'string', required: true },
date: { type: 'date', required: true },
author: { type: 'string', required: true }
}
}))
Full MDX blog in 5 minutes
Shipixen creates and sets up a fully functional MDX blog in minutes, with custom themes and SEO-friendly blog posts.To keep things organized, structure your content files like this:
Directory | Purpose | Content Type |
---|---|---|
/content/posts | Blog articles | Long-form MDX content |
/content/snippets | Code examples | Technical MDX snippets |
/content/docs | Documentation | Reference material |
For dynamic content, consider integrating a headless CMS alongside your MDX setup.
Steps for Headless CMS Integration
Adding a headless CMS allows for more dynamic content management beyond static files. Here's how to get started:
Define Content Models
Create clear and consistent content types to ensure reliable data handling:
// lib/content/types.ts interface ContentType { title: string; slug: string; content: string; metadata: { author: string; publishDate: Date; tags: string[]; } }
Fetch Content Dynamically
Write functions to retrieve content from your CMS:
// lib/content/fetch.ts async function getContent(slug: string) { const content = await fetch(`/api/content/${slug}`) return content.json() }
Optimize with Caching
Implement caching to improve performance and reduce unnecessary API calls.
These steps help you build a more dynamic and scalable content system.
Combining Static and Dynamic Content
Using both static MDX files and a headless CMS creates a flexible content strategy. Here's how they complement each other:
Content Type | Use Case | Benefits |
---|---|---|
Static MDX | Blogs, documentation | Quick loading, version control |
Dynamic CMS | News, updates | Real-time updates, collaboration |
Hybrid Content | Product pages | Combines flexibility and control |
For example, you can use a hybrid approach in your Next.js application:
// pages/[type]/[slug].tsx
export async function getStaticProps({ params }) {
const content = params.type === 'blog'
? await getMDXContent(params.slug)
: await getCMSContent(params.slug)
return {
props: { content },
revalidate: 60 // Update content every minute
}
}
If you're looking for a ready-to-use solution, platforms like Shipixen offer pre-configured setups. These setups include SEO-friendly blogs built with MDX, support for search functionality, tags, code blocks, multiple authors, and more. This streamlines development while accommodating diverse content needs.
Managing Content Updates
Effectively managing content updates in Next.js requires a structured workflow to ensure consistency and accuracy. This section covers strategies for handling content changes, focusing on version control and streamlined update processes.
Content Version Control
Next.js doesn’t include built-in versioning, but its flexible data-fetching capabilities allow you to implement custom solutions. Below is an example of how to define and retrieve versioned content:
// lib/content/version.ts
interface ContentVersion {
id: string;
content: string;
version: number;
updatedAt: Date;
status: 'draft' | 'published' | 'archived';
}
async function getContentVersion(slug: string, version?: number) {
const content = await prisma.content.findFirst({
where: {
slug,
version: version || undefined,
status: 'published'
},
orderBy: { version: 'desc' }
});
return content;
}
By implementing this type of version control, you can track content updates with precision, ensuring every change is documented.
Version Control Options
Various content types require tailored approaches to versioning. Here’s a breakdown:
Content Type | Version Control Method | Update Frequency |
---|---|---|
Static Pages | Git-based versioning | Low (weekly/monthly) |
Dynamic Content | Database versioning | High (daily) |
User-generated | Revision history | Real-time |
Configuration | Environment variables | Per deployment |
For example, you can track content changes in a database using the following method:
// lib/content/tracking.ts
async function trackContentChanges(contentId: string, changes: any) {
await prisma.contentHistory.create({
data: {
contentId,
changes: JSON.stringify(changes),
timestamp: new Date(),
userId: getCurrentUser().id
}
});
}
Once versioning is set up, you can integrate it into a structured update process for better content management.
Content Update Process
Using version tracking as a foundation, follow these three steps to manage content updates efficiently:
Draft Creation
Create a draft version of your content for review:
// pages/api/preview/[slug].ts export default async function preview(req, res) { const { slug } = req.query; if (!slug) { return res.status(400).json({ message: 'Missing slug parameter' }); } res.setPreviewData({ slug, version: 'draft' }); res.redirect(`/content/${slug}`); }
Approval Workflow
Submit the draft for review to ensure quality control:
// lib/content/review.ts interface ReviewStatus { approved: boolean; reviewerId: string; comments: string[]; reviewDate: Date; } async function submitForReview(contentId: string) { return await prisma.review.create({ data: { contentId, status: 'pending', submittedAt: new Date() } }); }
Publication Process
Publish the content once it’s approved:
// lib/content/publish.ts async function publishContent(contentId: string) { const review = await getLatestReview(contentId); if (!review.approved) { throw new Error('Content must be approved before publishing'); } return await prisma.content.update({ where: { id: contentId }, data: { status: 'published', publishedAt: new Date() } }); }
If you’re using Shipixen’s content management system, its built-in MDX blog features can simplify this process. Shipixen supports draft previews, scheduled publishing, and content rollbacks, eliminating the need for custom setups.
Content Management Guidelines
Use a component-based architecture in Next.js to improve scalability, simplify updates, and make content management more efficient.
Component-Based Content Structure
Here's an example of a reusable content component:
// components/content/ArticleCard.tsx
interface ArticleProps {
title: string;
excerpt: string;
author: Author;
publishDate: Date;
}
export function ArticleCard({ title, excerpt, author, publishDate }: ArticleProps) {
return (
<article className="content-card">
<h2>{title}</h2>
<p>{excerpt}</p>
<footer>
<AuthorBio author={author} />
<time>{formatDate(publishDate)}</time>
</footer>
</article>
);
}
Why use this approach?
Benefit | How It Works | Result |
---|---|---|
Reusability | Shared components | Faster development |
Consistency | Standardized elements | Uniform branding |
Maintainability | Isolated updates | Easier fixes |
Performance | Optimized rendering | Faster page speeds |
Core Technical Requirements
To ensure your content management system performs well, adhere to these key technical practices:
Accessibility Standards
Make sure your components comply with WCAG 2.1 guidelines:
// Ensure accessible content export function RichText({ content }: { content: string }) { return ( <div role="article" className="prose" aria-label="Article content" > <MDXRenderer>{content}</MDXRenderer> </div> ); }
Performance Optimization
Optimize how content is loaded and displayed:
// Efficient content loading export async function loadContent(slug: string) { const content = await contentLoader.load(slug); return { ...content, optimizedImages: await generateOptimizedImages(content.images), cacheControl: 'public, max-age=3600' }; }
SEO Configuration
Improve search engine visibility with proper metadata:
// SEO-friendly metadata export async function generateMetadata({ params }: Props) { const article = await getArticle(params.slug); return { title: article.title, description: article.excerpt, openGraph: { type: 'article', publishedTime: article.publishDate } }; }
These practices ensure your content system is accessible, efficient, and optimized for search engines.
Using Shipixen for Content Projects
Shipixen simplifies Next.js content management by offering a pre-configured MDX blog with SEO-friendly features.
In minutes, you have everything set up plus
- all SEO, JSON-LD, and Open Graph automatically generated
- a fully functional MDX blog with search, tags, and authors
- a component library with 100s of examples
Conclusion
Main Points Review
Creating an efficient content management system in Next.js requires a focus on scalability and ease of maintenance. Here are the core components to keep in mind:
Component | Purpose | Benefit |
---|---|---|
Component Architecture | Modular content blocks | Speeds up development |
Dynamic Routing | Organizes content logically | Improves user navigation |
Version Control | Manages content changes | Ensures better governance |
SEO Optimization | Boosts search rankings | Increases organic traffic |
These elements combine to form a strong foundation for a content management system that can grow alongside your project. With modern tools, managing content in Next.js has become more straightforward and efficient, offering a practical way to meet evolving project needs.
Implementation Guide
Taking these principles further, here's a practical guide to building your Next.js content management system:
Initial Setup and Configuration
Start by setting up a solid base using Next.js's powerful features. Tools like Shipixen's codebase generator can simplify the process. It supports Next.js 15, TypeScript, and Tailwind CSS, and includes pre-configured features like SEO, sitemaps, and MDX blog support [1].
Content Structure Development
Use an MDX-based blog structure for a lightweight, performant and SEO-friendly blog. No databases means no maintenance headaches.
Content management isn't just about organizing and displaying information - it's about building a system that can evolve with your project. By following these steps and using the right tools, you can create a system that works well today and adapts to future needs.
FAQs
How can integrating a headless CMS improve content management in Next.js?
Integrating a headless CMS with Next.js can significantly enhance your content management workflow by separating content creation from its presentation. This decoupled approach allows developers to focus on building dynamic, scalable frontends while content editors can manage and update content independently through an intuitive interface.
With a headless CMS, you can easily implement features like content versioning, seamless updates, and structured data management. Combining this with Next.js's performance optimizations, such as server-side rendering (SSR) and static site generation (SSG), ensures faster load times and a better user experience. This setup is ideal for teams looking to streamline collaboration and deliver high-quality digital experiences efficiently.
What advantages does MDX offer for content creation in Next.js, and how is it different from traditional Markdown?
MDX combines the simplicity of Markdown with the power of JSX, allowing you to seamlessly include React components within your content. This makes it ideal for building dynamic, interactive pages in Next.js. Unlike traditional Markdown, which is limited to static content, MDX enables you to embed functionality, such as custom UI components or data-driven elements, directly into your content files.
By using MDX in Next.js, you can streamline your workflow, maintain a consistent design system, and create more engaging user experiences, all while keeping your content easy to manage and update.
What are some effective strategies for managing content versioning and updates in a Next.js project?
Efficient content versioning and updates in a Next.js project can be achieved by implementing a few key strategies:
- Use a version control system (like Git) to track changes in your codebase and content files. This ensures you can easily roll back changes or collaborate with your team.
- Leverage incremental static regeneration (ISR) in Next.js to update specific pages without rebuilding the entire site, improving performance and deployment speed.
- Organize content with a headless CMS or structured file system, making it easier to manage, update, and version content independently of your code.
By combining these approaches, you can streamline your content management process and maintain a scalable, efficient workflow for your Next.js projects.
Table of Contents
Authors
- Name
- Shipixen
Previous Article
Next Article
Table of Contents
Authors
- Name
- Shipixen