- Updated on
Next.js File Naming Best Practices
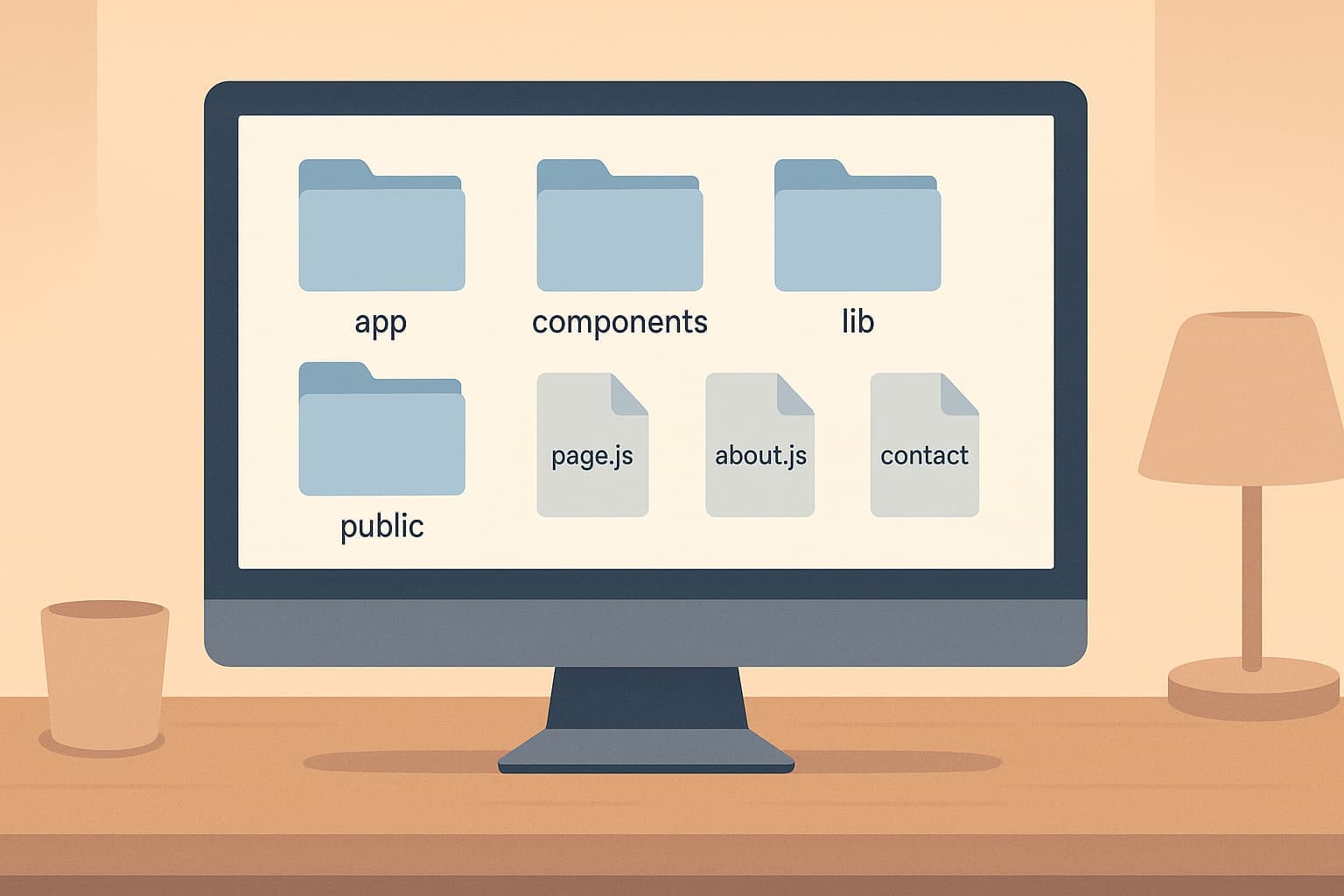
Proper file naming in Next.js is crucial for maintaining an organized, scalable, and easy-to-navigate codebase. Here's what you need to know:
- Key Files for Routing: Use
page.js
for routes,layout.js
for shared layouts,error.js
for custom errors, andloading.js
for loading states. - Naming Conventions:
- File Names & React Components: Use
kebab-case
for compatibility and readability (e.g.,user-profile.tsx
). - Variables and Props: Stick to
camelCase
(e.g.,userSettings
).
- File Names & React Components: Use
- Folder Organization:
- Group files by feature for better scalability (e.g.,
features/auth/components/login-form.tsx
).
- Group files by feature for better scalability (e.g.,
- Dynamic and Catch-All Routes:
- Use
[param]
for dynamic routes and[...param]
for catch-all routes.
- Use
Quick Comparison of Naming Patterns
Pattern | Use Cases | Benefits | Drawbacks |
---|---|---|---|
PascalCase | React component names, interfaces, class names | Easy to identify components | Slightly harder to type |
camelCase | Variables, props, functions | Standard for JavaScript | Harder to read with long words |
kebab-case | File names, URLs, CSS classes | Readable, URL-friendly | Requires conversion for JS |
Organizing your files and sticking to these conventions ensures your Next.js project is easier to manage, debug, and scale over time.
Next JS Project Structure: Patterns and Techniques for Success
Next.js File Naming Rules
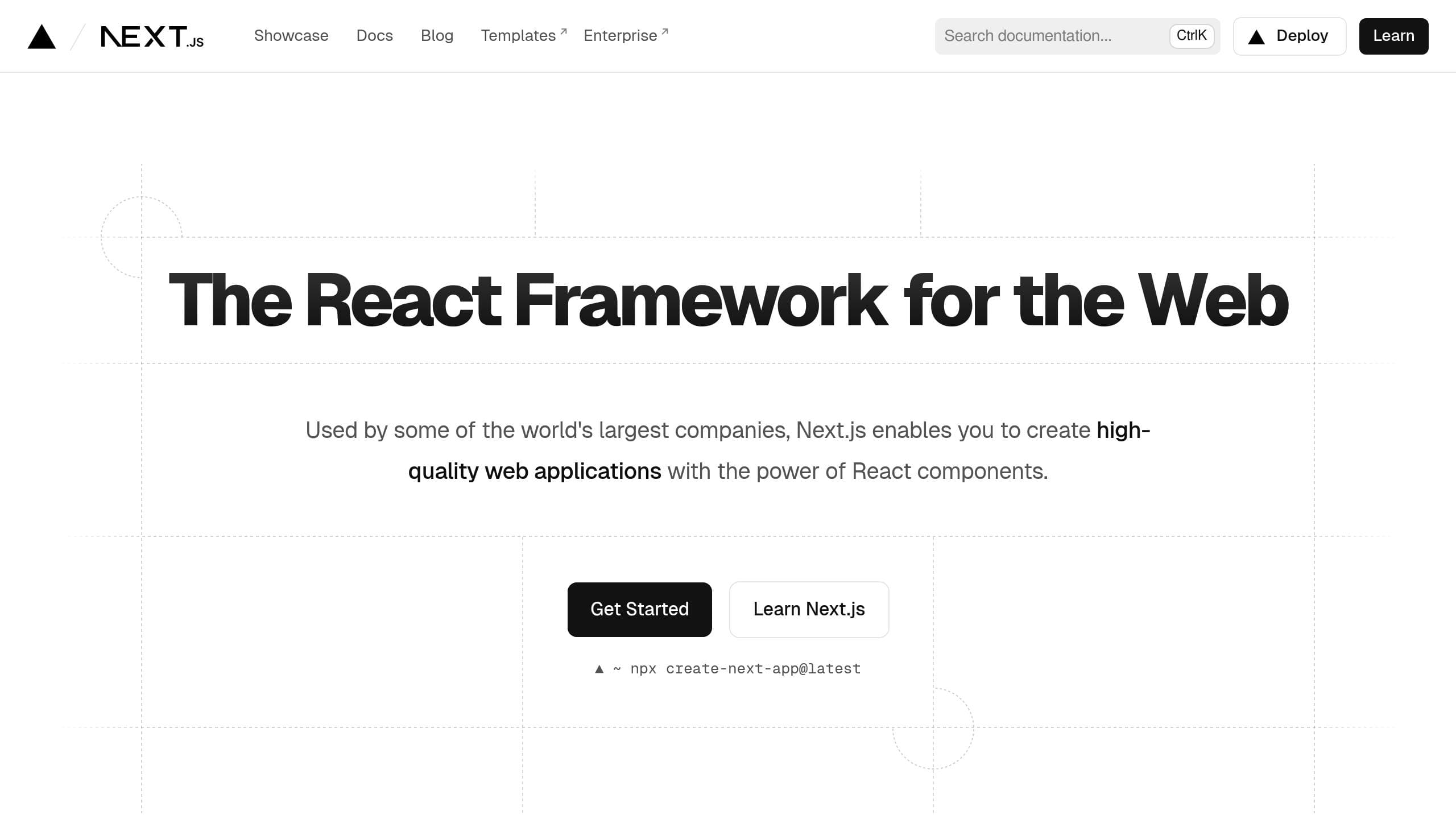
Next.js uses specific file naming conventions to define your app's routing and structure, helping keep everything organized.
File and Component Naming Standards
Sticking to Next.js routing conventions, having a clear and consistent naming strategy makes your project easier to scale and maintain.
File Names
Use kebab-case for file names to ensure compatibility across operating systems and URLs:
├── components/
│ ├── user-profile.tsx
│ ├── product-card.tsx
│ └── navigation-menu.tsx
Keep file names short yet descriptive, stick to kebab-case, and avoid special characters except for hyphens. Always use the correct file extensions.
Component Names
React component names should follow PascalCase, where each word starts with a capital letter. This helps differentiate components from standard functions or HTML elements:
UserProfile.tsx
export default function UserProfile() {
return (
<div className="user_profile_container">
<h1>User Profile</h1>
</div>
);
}
This approach ensures your code remains clear and easy to follow.
Naming Pattern Rules
Stick to these conventions throughout your project:
Element Type | Naming Convention | Example |
---|---|---|
Files | kebab-case | auth-provider.tsx |
Component names | PascalCase | AuthProvider |
Props | camelCase | userSettings |
Custom Hooks | camelCase with 'use' prefix | useAuthState |
For TypeScript interfaces and types, use PascalCase with a descriptive prefix:
interface IUserProps {
firstName: string;
lastName: string;
}
type TAuthState = {
isAuthenticated: boolean;
user: IUserProps;
}
Additionally, adhere to Next.js defaults for special files in the app directory.
Get a professional codebase in minutes
Shipixen creates and sets up a fully functional codebase with all the best practices in place.Folder Organization Methods
A well-structured folder setup goes hand in hand with clear file naming to make your Next.js project easier to manage. A thoughtful folder organization improves navigation and simplifies maintenance as your project grows.
Feature-Based Folders
Group related files into feature-based folders to keep your code organized and focused. This method helps isolate functionality, making it easier to manage and scale:
src/
├── features/
│ ├── auth/
│ │ ├── components/
│ │ │ ├── login-form.tsx
│ │ │ ├── login-form.test.tsx
│ │ │ ├── signup-form.tsx
│ │ │ └── signup-form.test.tsx
│ │ ├── hooks/
│ │ │ ├── use-auth.ts
│ │ │ └── use-auth.test.ts
│ │ └── utils/
│ │ ├── auth-helpers.ts
│ │ └── auth-helpers.test.ts
This structure ensures that related code stays together, making it easier to:
- Find specific files or functionality
- Manage dependencies between components
- Scale individual features without affecting others
- Maintain clear boundaries between different parts of the application
A solid folder structure reinforces your project's overall architecture.
Grouping Routes for Better Readability
Route groups in Next.js offer a powerful way to organize your application without affecting the URL structure. Create route groups by wrapping a folder name in parentheses like (folderName)
:
app/
├── (marketing)/
│ ├── about/
│ │ └── page.tsx
│ ├── blog/
│ │ └── page.tsx
│ └── layout.tsx
├── (shop)/
│ ├── cart/
│ │ └── page.tsx
│ ├── products/
│ │ └── page.tsx
│ └── layout.tsx
└── (auth)/
├── login/
│ └── page.tsx
├── register/
│ └── page.tsx
└── layout.tsx
What makes route groups particularly useful:
- Logical organization without URL impact - group related routes by feature, team, or section
- Separate layouts for different sections of your app - each group can have its own layout.tsx
- Cleaner codebase structure - easily identify which routes belong together
- Team collaboration - different teams can work on separate route groups
- Simplified navigation - quickly find related routes in your project
Important considerations:
- Route groups don't affect URL paths -
(marketing)/about/page.tsx
still resolves to/about
- Avoid duplicate URL paths across groups -
(marketing)/about/page.tsx
and(shop)/about/page.tsx
would both resolve to/about
and cause an error - When using multiple root layouts without a top-level layout.js, define your home page in one of the route groups
- Navigation between different root layouts causes a full page load rather than a client-side navigation
Project Navigation
For larger codebases, a clear folder hierarchy is essential. Here's an example structure for organizing routes, components, and utilities:
src/
├── app/
│ ├── (auth)/
│ │ ├── login/
│ │ └── register/
│ ├── (dashboard)/
│ │ ├── analytics/
│ │ └── settings/
│ └── (marketing)/
│ ├── about/
│ └── contact/
├── components/
│ ├── ui/
│ └── shared/
└── lib/
├── utils/
└── config/
Key advantages include:
- Logical route grouping using parentheses without affecting URLs
- Separation of components from business logic
- Clear relationships between dependencies
Naming Patterns: Benefits and Drawbacks
Expanding on file and component naming standards, these naming patterns help improve your project's organization. Using the right naming patterns makes your code easier to read, maintain, and scale.
Pattern Comparison
Different naming patterns serve different purposes. Here's a detailed breakdown of the most common ones:
Pattern | Use Cases | Benefits | Drawbacks |
---|---|---|---|
PascalCase | React component names, interfaces, class names | • Identifies React components easily • Aligns with React's conventions • Visually distinct | • Slightly harder to type • May conflict with specific build systems |
camelCase | JavaScript variables, functions, props | • Standard for JavaScript • Easy to write and read • Works naturally for object properties | • Harder to read with long words • Can obscure word boundaries |
kebab-case | File names, URLs, CSS classes | • URL-friendly • Highly readable • Compatible with all systems | • Requires conversion for JavaScript • Needs extra handling during imports |
snake_case | Constants | • Separates words clearly • Works well for long identifiers • Platform-agnostic | • Rarely used in React projects • Takes up more space visually |
Consistently applying these patterns simplifies your codebase and reduces mental effort when navigating or updating files.
Example Usage
Here's how these patterns look in practice:
// PascalCase for component names
export default function UserProfile() {
return <div>Profile Content</div>;
}
// camelCase for props and functions
interface UserProfileProps {
firstName: string;
handleSubmit: () => void;
}
// File structure using kebab-case and snake_case
app/
├── (auth)/
│ └── login/
│ └── page.tsx
components/
├── user
│ └── user-profile.tsx
Conclusion
Using clear and consistent file naming in Next.js projects lays the groundwork for a codebase that's easy to manage and expand. When teams stick to well-defined naming conventions, they simplify collaboration and speed up the development process.
Here's why it matters:
- Makes navigating and maintaining code easier
- Helps new team members get up to speed quickly
- Cuts down on technical debt over time
Adopting patterns like kebab-case for routes and file names ensures your project stays organized as it grows. This predictability becomes even more valuable as your application scales. Tools like Shipixen can take this a step further by automating your setup, applying these conventions from the start, and letting your team focus on building features instead of worrying about structure.
FAQs
Why should I follow naming conventions like kebab-case or PascalCase in a Next.js project?
These conventions make it easier for developers to understand the structure of the codebase and reduce the chances of errors caused by inconsistent naming.
For example, kebab-case is commonly used for file and folder names because it is URL-friendly and easy to read, while PascalCase is ideal for React components as it aligns with JavaScript conventions for class names. By following these practices, your project will be more organized and easier to collaborate on, especially as it grows in complexity.
How does organizing folders by feature improve the scalability and maintainability of a Next.js project?
Organizing folders by feature in a Next.js project helps keep your codebase structured and intuitive, especially as the application grows. By grouping all related components, pages, and utilities for a specific feature into a single folder, you make it easier for developers to locate, update, and manage code without confusion.
Table of Contents
Authors
- Name
- Shipixen
Previous Article
Next Article
Table of Contents
Authors
- Name
- Shipixen